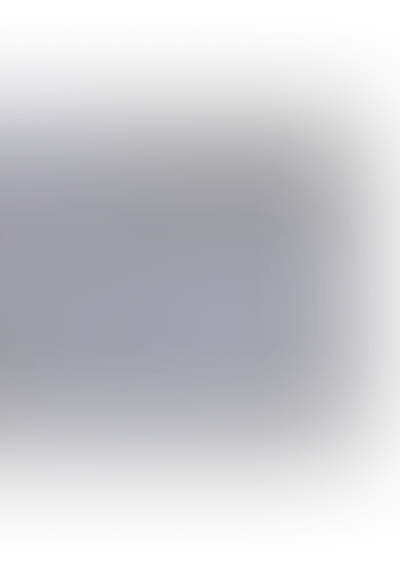
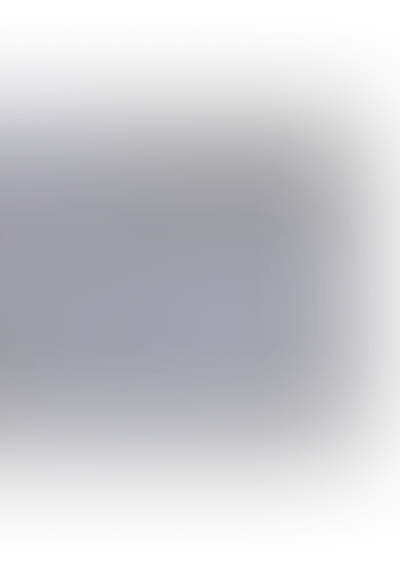
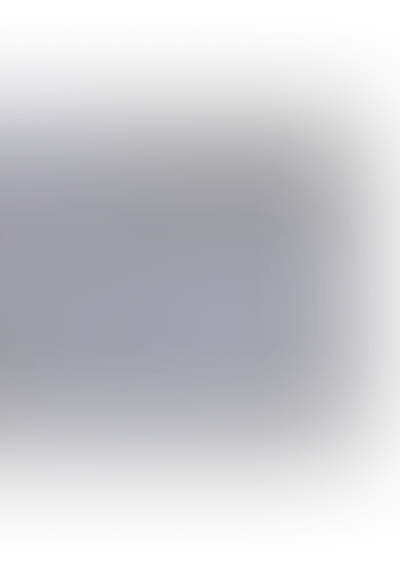
1
EdgeDB
1
EdgeQL
1
EdgeQL
Before we proceed ensure you have the following installed on your system:
- node
- npm
- edgedb
Project setup
First, we need to create a project. We will do this by using the
1
npm init
In terminal type :
1
npm init -y
Next, we need to install additional modules:
1
express
1
apollo-server-express
1
edgedb
1
cors
1
graphql
1
npm i express apollo-server-express edgedb cors graphql
We also need to initialize
1
EdgeDB
1
edgedb project init
Declaring EdgeDB schema
Edit
1
dbschema/default.esdl
1 2 3 4 5 6 7
module default { type TodoList { required property name -> str; property finished -> bool; property description -> str; } }
Then, run these two commands to migrate database
1 2
edgedb migration create edgedb migrate
Setting up a server
First, we will create
1
app.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
const { ApolloServer } = require("apollo-server-express") const cors = require("cors") const express = require("express") const { typeDefs, resolvers } = require('./schema') const server = new ApolloServer({ typeDefs, resolvers }) const app = express() app.use(cors()) server.start().then(() => server.applyMiddleware({ app })) const port = process.env.PORT || 3000 app.listen({ port }, () => console.log(`Server is up and running on port ${port}`))
It's also required to create
1
schema.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48
const { gql } = require("apollo-server-express") const todoList = require("./todoList") const resolvers = { Query: { getTodoList: async (_, ) => await todoList.getAll(), getTodoItem: async (_, { id }) => await todoList.getById(id) }, Mutation: { createTodoItem: async (_, { payload }) => await todoList.create(payload), updateTodoItem: async (_, { payload }) => await todoList.update(payload), deleteTodoItem: async (_, { id }) => await todoList.deleteById(id) } } const typeDefs = gql` input CreateTodoItemInput { name: String! finished: Boolean description: String } input TodoListInput { id: ID! finished: Boolean! } type Query { getTodoList: [TodoList] getTodoItem(id: ID!): TodoList } type Mutation { createTodoItem(payload: CreateTodoItemInput!): Boolean updateTodoItem(payload: TodoListInput!): Boolean deleteTodoItem(id: ID!): Boolean } type TodoList { id: ID name: String description: String finished: Boolean } ` module.exports = { typeDefs, resolvers }
We are also going to create
1
todoList.js
1
EdgeDB
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
const edgedb = require("edgedb"); const client = edgedb.createClient() const getAll = () => { return client.query(`SELECT TodoList { name, description, finished }`) } const getById = (id) => { return client.querySingle(`SELECT TodoList { name, description, finished } FILTER .id = <uuid>$id`, { id }) } const create = async (payload) => { let result = await client.querySingle(`INSERT TodoList { name := <str>$name, finished := <bool>$finished, description := <str>$description }`, { name: payload.name, finished: !!payload.finished, description: payload.description || "" }) return !!result } const update = async (payload) => { let result = await client.querySingle(`UPDATE TodoList FILTER .id = <uuid>$id SET { finished := <bool>$finished}`, { id: payload.id, finished: !!payload.finished }) return !!result } const deleteById = async (id) => { let result = await client.querySingle(`DELETE TodoList FILTER .id = <uuid>$id`, { id }) return !!result } module.exports = { getAll, getById, create, update, deleteById }
Congratulations, you've just created Node.js/Graphql/EdgeDB project!