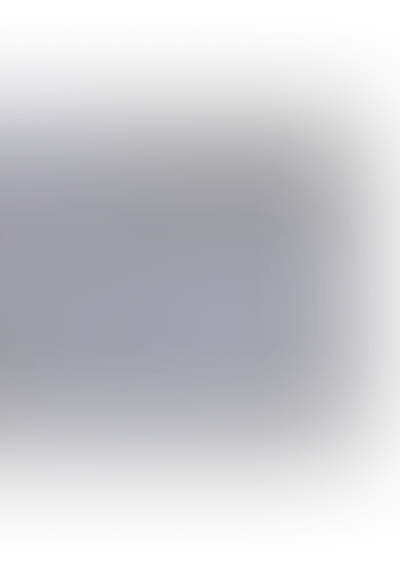
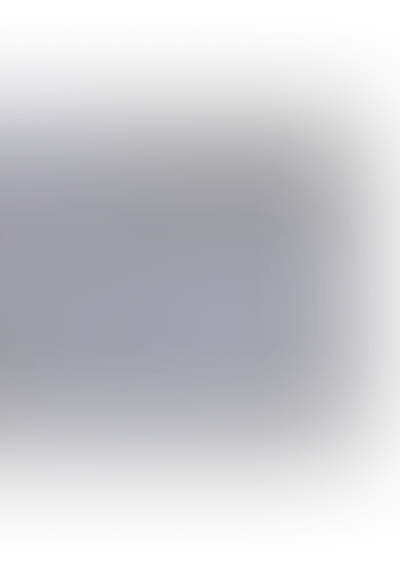
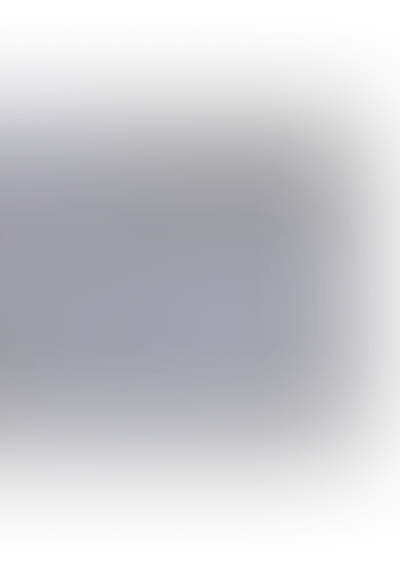
Next.js provides server-side rendering for React applications. In this post, we will learn how to translate the text in a Next.js app by using
1
next-translate
Before we proceed ensure you have the following installed on your system:
- node
- npm
- yarn
Project setup
First, we need to create a project. We will do this by using the
1
create-next-app
In terminal type :
1
npx create-next-app
This will run an interactive prompt that will ask for the project name. You can name it whatever you like. For the sake of this post, we named ours
1
next-translate
1
yarn dev
1
localhost:3000
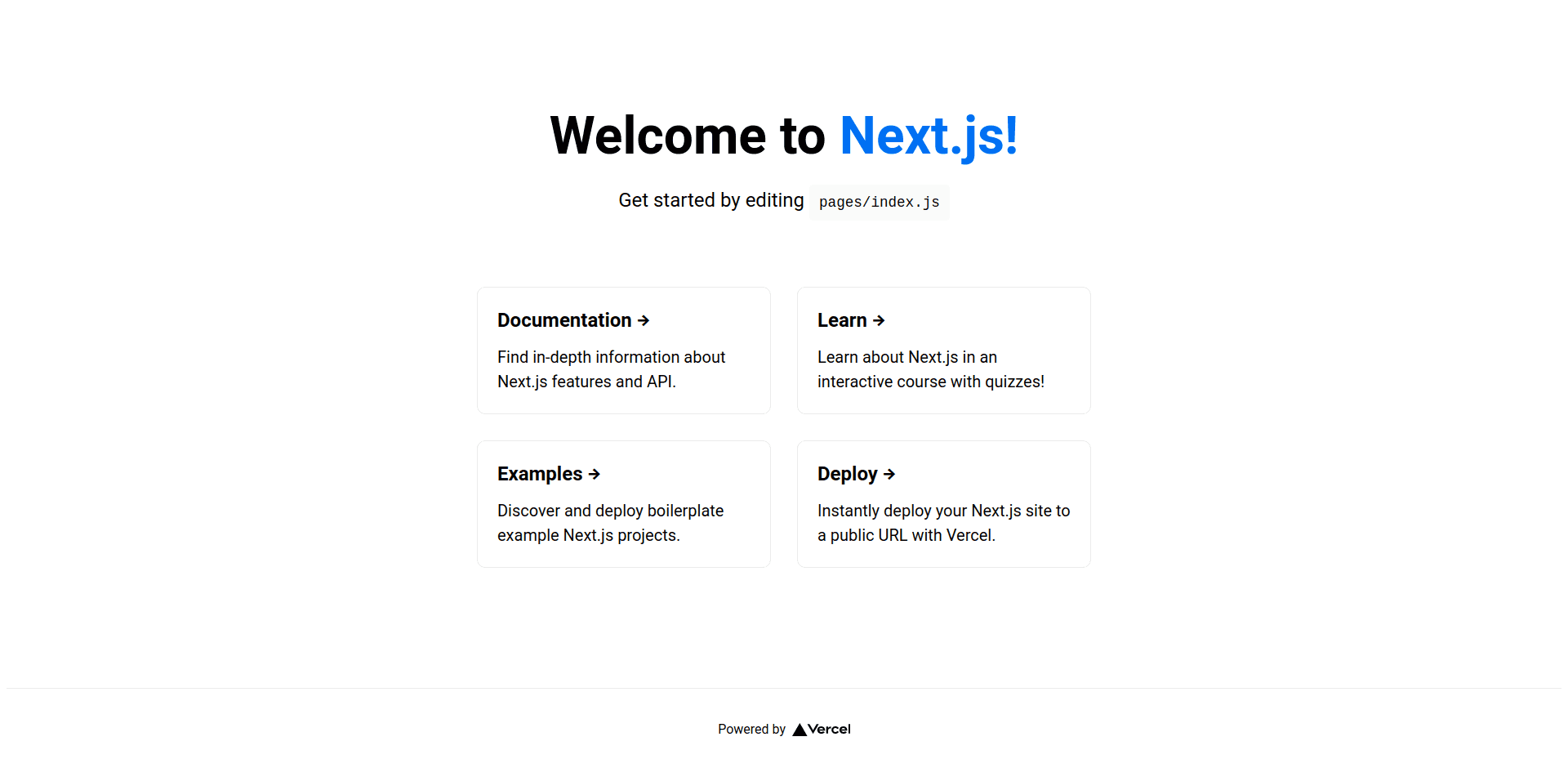
We don't need these components and pages from the starter template so now you'll need to delete the
1
styles/Home.module.css
1
api
1
pages
1
pages/index.js
1 2 3 4 5 6 7
const Home = () => { return ( <h1>Home</h1> ) } export default Home
We will also have an
1
about
1 2 3 4 5 6 7
const About = () => { return ( <h1>About</h1> ) } export default About
After getting this done we need to install a library for translating our pages. Open up a terminal in your project directory and type
1
yarn add next-translate
Translations
First, we need to create
1
next.config.js
1 2 3
const nextTranslate = require('next-translate') module.exports = nextTranslate()
After that, we need to add the
1
i18n.json
1
next-translate
1 2 3 4 5 6 7 8
{ "locales": ["en", "me"], "defaultLocale": "en", "pages": { "/": ["home"], "/about": ["about"] } }
- In the array we specify all the locales that we want to use in our project (uses ISO format)
1
locales
- is required so that
1
defaultLocale
knows what is our default language (uses ISO format)1
next-translate
- In we specify namespaces used in each page. To add namespaces to all pages use
1
pages
{"*": ["common"]}1
(eg:
/locales` root directory.1
). You can also use regex to specify what locales are used in pages. \ \ After creating configuration files we now need to specify namespaces. By default, they are specified inside the
1 2 3 4 5 6
├── en │ ├── about.json │ └── home.json └── me ├── about.json └── home.json
In these
1
.json
1
i18n.json
1
en/home.json
1 2 3 4 5
{ "title": "Home Page", "description": "This is a home page description written in the English language.", "current-language": "Current locale is set to /{{language}}" }
You probably noticed
1
{{language}}
1
useTranslation
1
next-translate/useTranslation
1
.json
1
/locales
1
index.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
import useTranslation from "next-translate/useTranslation" const Home = () => { const { t, lang } = useTranslation("home") const title = t("title") const description = t("description") const language = t("language", { language: lang }) return ( <> <h1>{title}</h1> <h4>{description}</h4> <p>{language}</p> </> ) } export default Home
Let's explain line by line what is happening here.
By using the
1
useTranslation
1
t
1
lang
1
t
1
lang
By adding a parameter to the
1
useTranslation
1
next-translate
1
i18n.json
1
root
1
t("title")
1
/locales/(en/me)/home.json
By using
1
t("language", { language: lang })
1
language
Routing to different locales
From version
1
10
1
Internationalized Routing
Let's say that you have 2 different locales
1
en
1
me
1
Link
1
next/link
Link component
In NextJS
1
Link
1 2 3 4 5
import Link from "next/link"; <Link href="/about"> <a>About</a> </Link>
but if we want to send the user to the
1
/about
1
locale
1
Link
1 2 3
<Link href="/about" locale="en"> <a>About</a> </Link>
This also means that
1
next-translate
As we can see, translation in NextJS is convenient, easy to set-up, and use. You can find the code by clicking here. It is a GitHub repository with the code from this article that you can use and modify. The project in this repository contains basic styling and routing.